How to programmatically count tokens BEFORE making a request to LLM model provider?
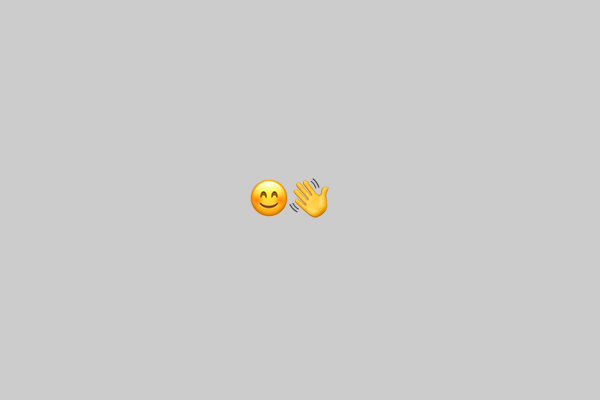
Programmatic Token Counting for LLM Model Providers
When working with Large Language Model (LLM) providers, it's essential to manage your token usage to avoid rate limiting or exceeding model limits. One way to do this is by programmatically counting tokens before making a request to the LLM model provider. Here's how you can achieve this:
Client-Side Token Counting with Transformers.js
- Use Transformers.js: Utilize the Transformers.js library, which provides a JavaScript implementation of popular transformer models.
- Leverage hosted pretrained models and WASM binaries: By default, Transformers.js uses hosted pretrained models and precompiled WASM binaries, which should work out-of-the-box.
- Token counting: Use the
tokenizer.encode()
method to count the tokens in your input text.
Example Code
Here's an example code snippet in JavaScript that demonstrates client-side token counting using Transformers.js:
import { AutoTokenizer } from 'transformers';
const tokenizer = new AutoTokenizer('xenova/xenova-base');
const inputText = 'This is an example input text.';
const tokenCount = tokenizer.encode(inputText).length;
console.log(`Token count: ${tokenCount}`);
Using Xenova's Token Counter
- Visit Xenova's token counter: Head to https://huggingface.co/Xenova to use their token counter tool.
- Enter your input text: Paste your input text into the token counter tool.
- Get the token count: The tool will provide an estimate of the token count for your input text.
Why Token Counting is Crucial for LLMs
- Gain extra understanding of LLMs: Token counting helps you understand how LLMs process input text, enabling you to craft more effective prompts and inputs.
- Optimize prompting: By counting tokens, you can optimize your prompts to elicit the desired response from the LLM, improving the overall quality of the output.
- Input and output analysis: Token counting allows you to analyze the input and output of LLMs, providing valuable insights into their behavior and limitations.
- Avoid rate limiting and model limits: Token counting helps you manage your token usage, avoiding rate limiting and model limits that can disrupt your application.
Benefits of Client-Side Token Counting
- Faster token counting: Client-side token counting is faster than server-side counting, as it eliminates the need for an additional API request.
- Improved user experience: By counting tokens on the client-side, you can provide a more seamless user experience and reduce the likelihood of rate limiting or model limit errors.
- Enhanced security: Client-side token counting reduces the amount of sensitive data sent to the server, enhancing security and protecting user data.
By incorporating client-side token counting into your application, you can effectively manage your token usage, gain a deeper understanding of LLMs, and ensure a smooth experience when working with LLM model providers.